This week I was updating some documentation in a Jupyter Notebook that had placeholders for credentials in it. This got me to thinking, I wonder if it is possible to get secrets from 1Password with PowerShell. 1Password as that is my password manager of choice.
A couple of quick searches later and I found the 1Password CLI that allows command-line access to a 1Password Vault. I also found a module that interfaces directly to a local 1Password instance and another that is an extension for the new PowerShell Secret Management module.
However, neither of these provided the functionality I was looking for. I wanted to be able to:
- Securely store locally a configuration for accessing a 1Password online Vault
- Automate authentication and retrieve a Session Token for interaction with a 1Password online Vault
- Automatically renew the Session Token if it expires
- Have multiple profile configurations for multiple 1Password Vaults
- Work in Windows PowerShell (5.x) and PowerShell (6.x/7.x + on Windows)
- Work in Jupyter Notebook
Introducing the 1Pwd PowerShell Module
My 1Pwd PowerShell Module is a wrapper for the 1Password CLI that allows full use of 1Password with PowerShell.
- Allows a configuration to be securely stored in your local Windows Profile that automatically loads with the module.
- Stores a profile configuration using Export-CliXML. The Export-Clixml cmdlet encrypts credential objects by using the Windows Data Protection API. The encryption ensures that only your user account on only that computer can decrypt the contents of the credential object. The exported CLIXML file can’t be used on a different computer or by a different user.
- You can then use the 1Password CLI commands without having to worry about Signing In and managing the Session Tokens.
- You can use the module in Demo’s and Presentations and not expose your API Keys or Credentials.
- Works in Jupyter Notebook
- Works with Windows PowerShell and PowerShell (6.x/7.x+ on Windows)
- Returns the full item from the Vault.
Here is an example of a Login Item for an online retailer
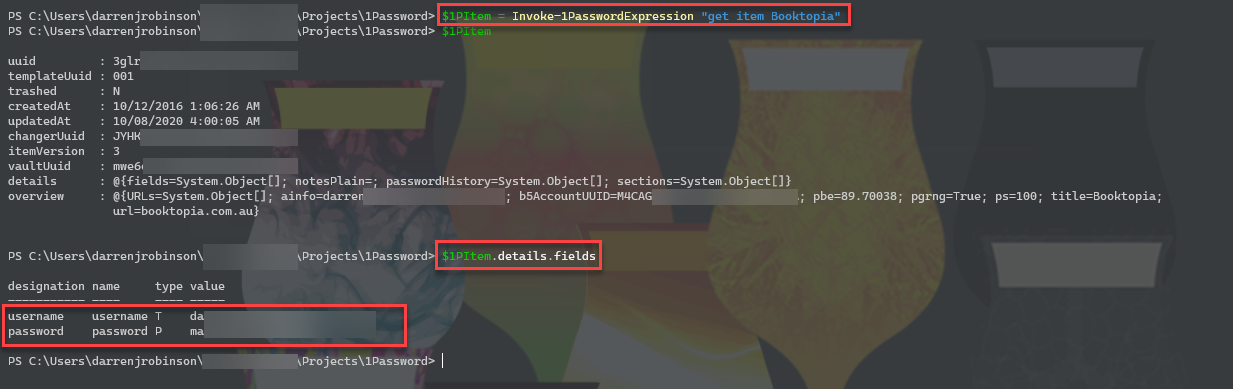
And an example of the module being used in a Jupyter Notebook.
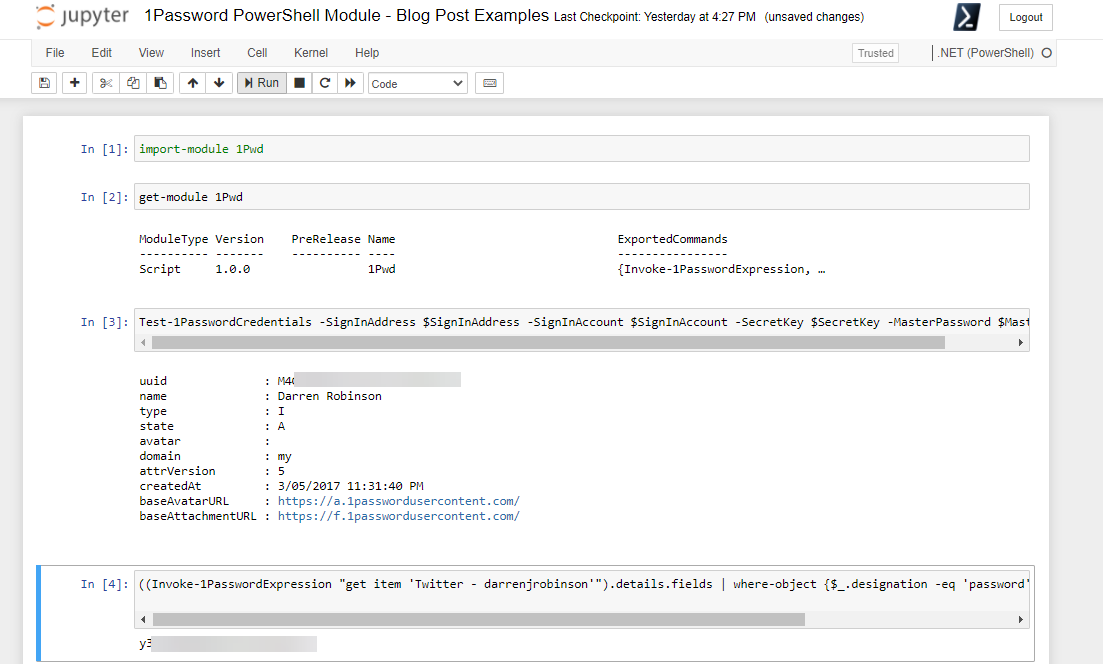
Prerequisites
To use this module you will need:
- A paid 1Password account
- Your 1Password Secret Key or Setup Code
- Your Master Password that you use for accessing your 1Password Vault
- 1Password CLI.
- Install it in your system path or in the same directory as the scripts you’ll be running that will use this 1Pwd PowerShell Module.
Installation
Install from the PowerShell Gallery on Windows PowerShell 5.1+ or PowerShell Core 6.x or PowerShell.
Install-Module -name 1Pwd
Test the 1Password CLI is accessible by running the following command that will return the 1Password CLI version. If you haven’t setup credentials yet you will also receive a message to that effect.
Invoke-1PasswordExpression "--version"

Configuration
Configuration involves creating a profile with the authentication information for a 1Password online Vault. You will need the Sign-In address, Email address, Secret Key and Master Password associated with your Vault. The Master Password is the password you type in to open your vault. You can get the rest of the required information from a local installation as shown below.
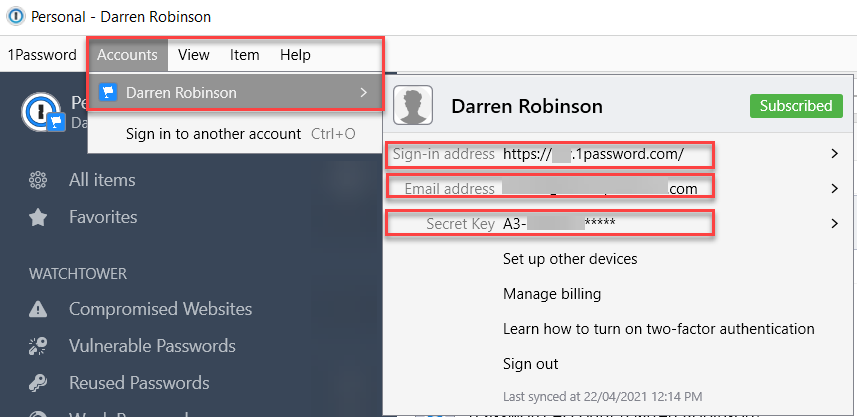
Or if you aren’t using the local Windows client you can get the information from your online vault after signing in.
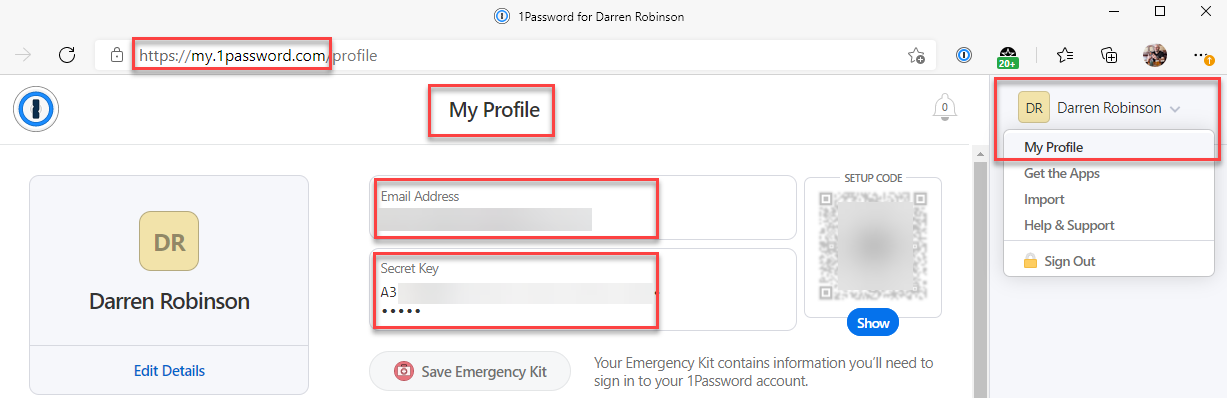
To create a secure profile for use with the 1Pwd Module execute the following PowerShell commands with the user account on the computer that you will be using to retrieve/set 1Password Vault items. This will create the secure configuration under your Windows Profile for the logged in user on computer it was executed on. It can only be opened and the Secret Key and Master Password read using the same account on the same computer.
Set Credentials and Profile Info
Update the following with your Sign-In Address and Sign-In Account (Email Address) retrieved above. You will be prompted to securely input your Secret Key and Master Password.
$1PSignInAddress = "https://my.1password.com"
$1PSignInAccount = "your@emailaddress.com"
$1PSecretKey = Read-Host "Enter your 1Password SecretKey" -AsSecureString
$1PMasterPassword = Read-Host "Enter your 1Password Master Password" -AsSecureString
Get 1Password Account Details
Using the information input above the Test-1PasswordCredentials cmdlet is used to validate them and return your account details.
$account = Test-1PasswordCredentials -SignInAddress $1PSignInAddress -SignInAccount $1PSignInAccount -SecretKey $1PSecretKey -MasterPassword $1PMasterPassword
Save 1Pwd Module Configuration
Having successfully provided and validated your credentials the Set-1PasswordConfiguration cmdlet will securely store the configuration in the logged in users local Windows Profile. When saving a configuration, you can use the -default switch to specify that it is the default configuration. It will automatically be retrieved and a session created when the module loads.
Set-1PasswordConfiguration -Vault $account.domain -SignInAddress $1PSignInAddress -SignInAccount $1PSignInAccount -SecretKey $1PSecretKey -MasterPassword $1PMasterPassword -Default
Using the 1Pwd Module
The primary command/cmdlet that you will use after configuration is Invoke-1PasswordExpression.
List Vaults
Invoke-1PasswordExpression "list vaults"

Get the Item Twitter
Invoke-1PasswordExpression "get item Twitter"

Get another item ‘Twitter Other Account’
e.g. An Item with spaces
Invoke-1PasswordExpression "get item 'Twitter - darrenjrobinson'"

Get the Twitter Vault Item and return just the password
((Invoke-1PasswordExpression "get item 'Twitter - darrenjrobinson'").details.fields | where-object {$_.designation -eq 'password'} | select-object -property value).value

Update an Item with a new user specified password
# Get Vault Entry $1PwdItem = Invoke-1PasswordExpression "get item '1PWD Module Update Test'" # Update the Item with new password 'mynewpassword' Invoke-1PasswordExpression "edit item $($1PwdItem.uuid) password=mynewpassword"
Update an Item with a new 1Password generated password and retrieve it and the old using Password History
# Get Vault Entry $1PwdItem = Invoke-1PasswordExpression "get item '1PWD Module Update Test'" # Update by getting 1Password to generate a 32-character password made up of letters, numbers, and symbols Invoke-1PasswordExpression "edit item $($1PwdItem.uuid) --generate-password" # Get Updated Item $updatedItem = Invoke-1PasswordExpression "get item $($1PwdItem.uuid)" # Get New Password $updatedItem.details.fields | where-object {$_.designation -eq 'password'} | select-object # Get Old Passwords $updatedItem.details.passwordHistory
Summary of other useful commands
Primarily I’ve written this to retrieve secrets from 1Password. The 1Password CLI is fully featured so allows everything you could do via a GUI client. Look at the documentation here for Creating, Deleting and Editing items, users, groups and documents in your 1Password vault.
Switch Vaults / Configuration
The Switch-1PasswordConfiguration cmdlet allows you to switch vaults/configuration. This is useful if you have multiple accounts. Each configuration needs to be saved using Set-1PasswordConfiguration. When saving a configuration, you can use the -default switch with Set-1PasswordConfiguration to specify which is the default configuration that will be loaded when the module loads.
To change to the configuration for PersonalVault2 you would use the command.
Switch-1PasswordConfiguration -vault PersonalVault2
To switch to the PersonalVault2 configuration and make it the default use the -default switch.
Switch-1PasswordConfiguration -vault PersonalVault2 -Default
Summary
Developing this module ended up taking a little longer than I originally anticipated, but I hope others may get some benefit from this.
The configuration profile component will only function on Windows. Potentially in the future someone could submit a Pull Request with changes to support other platforms.