Update: Oct 2019. Certification Campaigns can be easily managed using the SailPoint IdentityNow PowerShell Module.
This is the second post in the Certifications by API series. The last post detailed searching and retrieving campaigns. If you haven’t read that, check that out as it will give you the background for this one.
Also as per the last post this post also assumes you are authenticated to IdentityNow as detailed in this post, and you understand that this post details accessing Certifications using the non-versioned SailPoint IdentityNow API’s.
With that all said, this post details the creation of IdentityNow Certification Campaigns via the API using PowerShell. The Create Campaigns from IdentityNow Search process goes like this;
- using the Search API, find the users connected to a Source (or a group of users based on other criteria)
- iterate through them to identify their Role(s), Entitlement(s) and Source(s) and create a Manager Certification Campaign
- Specify the period for the Campaign along with options such as notifications and revocation
- Start the Campaign
Campaign Creation
As stated above the first task is to search and retrieve candidates for the campaign. This uses the Search function as I described in more detail in this post here.
If you have more than the searchLimit allowable via the API you will need to page the results over multiple queries. I’ll detail how to do that in a future post. In the query below I’m searching for users on the Source “Active Directory”.
$searchLimit = '2500' # Search Identities URI $searchURI = "https://$($orgName).api.identitynow.com/v2/search/identities?" # Query for Source that Campaign is for $query = '@accounts(Active Directory)' # Search Accounts $Accounts = Invoke-RestMethod -Method Get -Uri "$($searchURI)limit=$($searchLimit)&query=$($query)" -Headers @{Authorization = "Basic $($encodedAuth)" } write-host -ForegroundColor Yellow "Search returned $($accounts.Count) account(s)"
With the users returned we need to iterate through each and look at the users Entitlements, Roles and Access Profiles. We are creating a Manager campaign for these users for all of these (you can reduce the scope if required). The PowerShell snippet to do that looks like this.
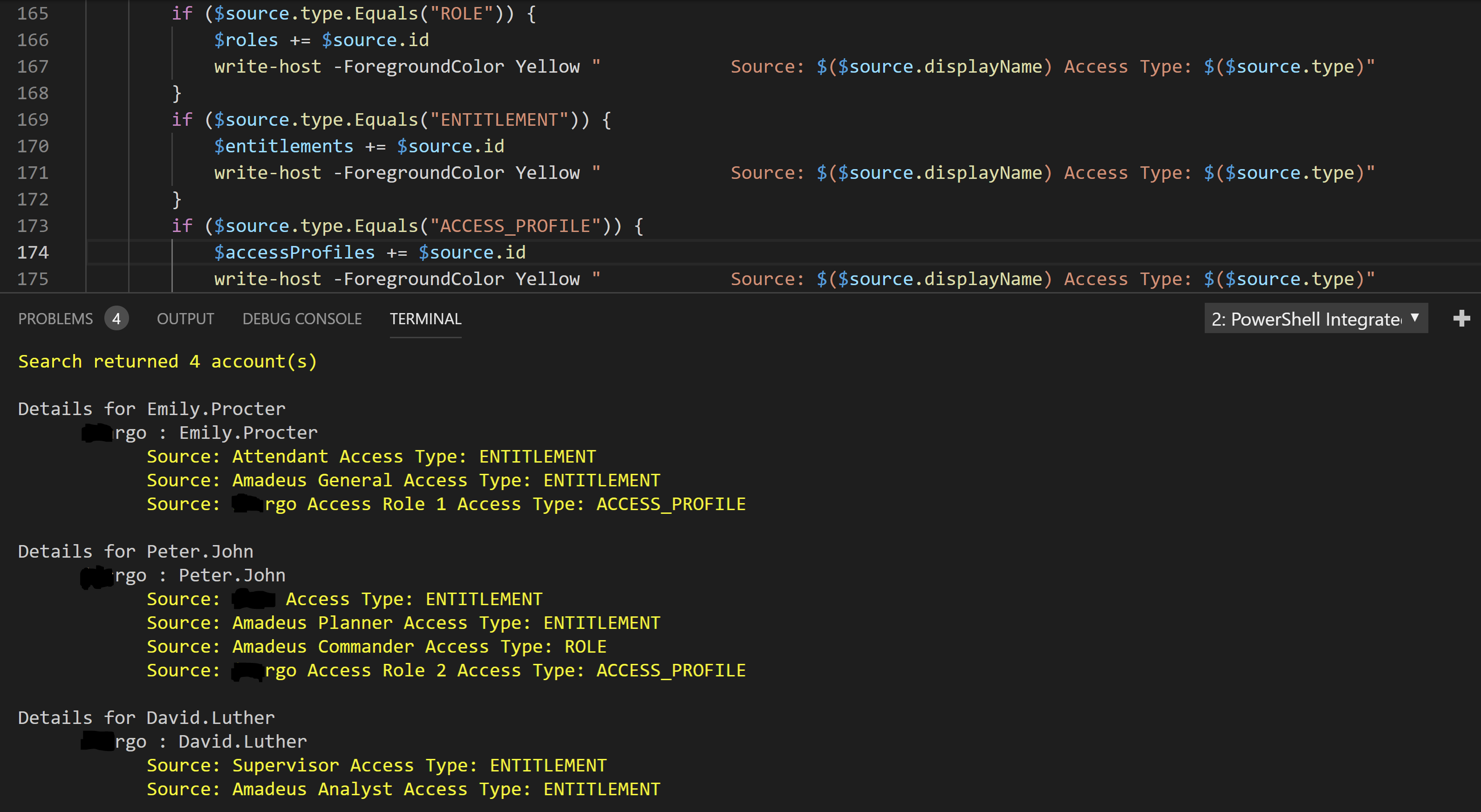
For inclusion in the campaign we need to build a collection for the Roles, Entitlements and Access Profiles. Using PowerShell to iterate through the list obtained from the users above therefore looks like this:
The summary after enumeration below shows the users for the source will be cover 1 Role, 9 Entitlements and 2 Access Profiles.
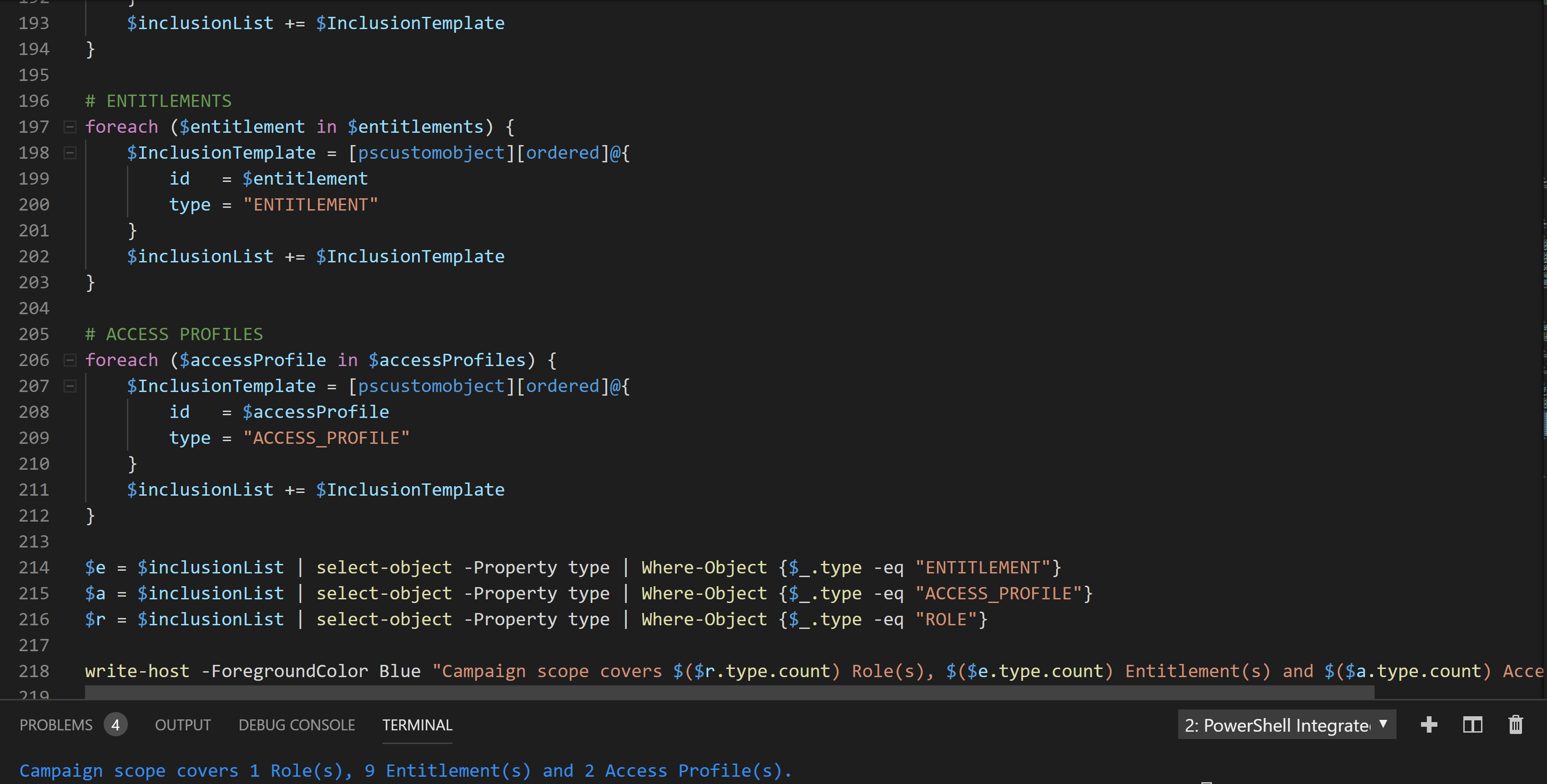
Now we have most of the information defined for the scope of our campaign we can specify the additional criteria and information such as duration, name, description, notification, and revocation. Each of those settings are self explanatory by the configuration setting. We then create the Campaign and and Activate it. I have a short delay after creation before activation as I found race conditions. You could lower the delay, but YMMV.
You can also add a Static Reviewer for the campaign as by default the owner will be the account you’re using to perform the creation. Add the following line into the configuration options and specify the ID for the identity.
$campaignOptions.Add("staticReviewerId", $reviewerUser.id )
The ID for an Identity can be obtained via Search. e.g.
# Get Campaign Reviewer in addition to the campaign creator $usrQuery = '@accounts Rick.Sanchez' $reviewerUser = Invoke-RestMethod -Method Get -Uri "$($searchURI)limit=$($searchLimit)&query=$($usrQuery)" -Headers @{Authorization = "Basic $($encodedAuth)" }
Putting it all together then looks like this in PowerShell.
The screenshot below shows the campaign being created.
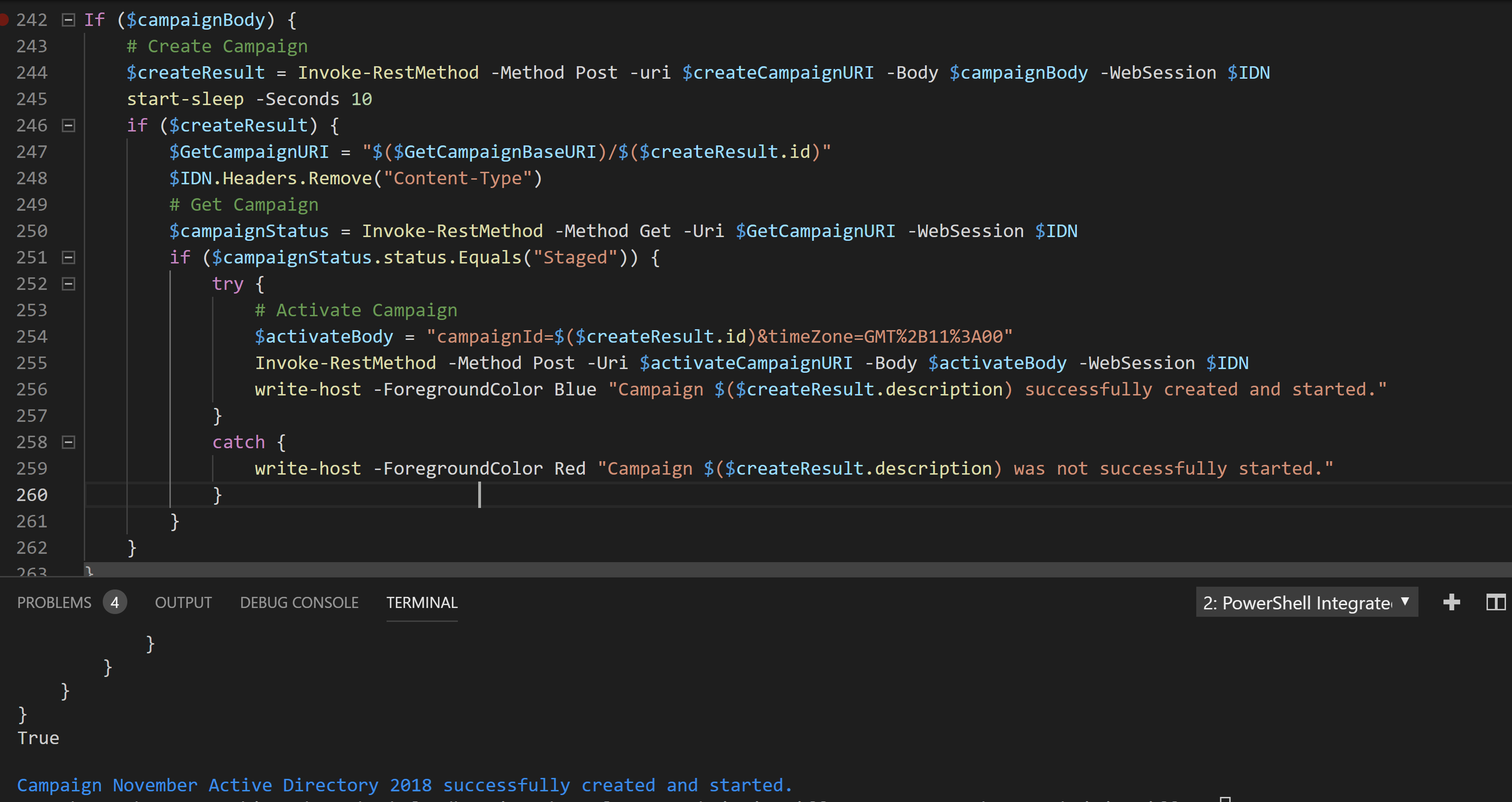
Looking at the Certifications section in the IdentityNow Portal we can see the newly created Campaign.
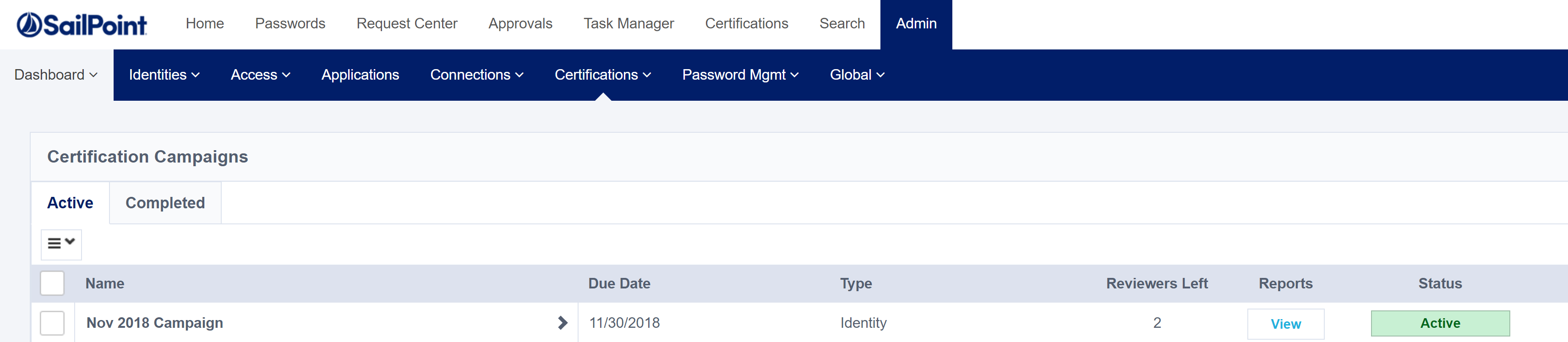
And we can see the two Managers requiring review.
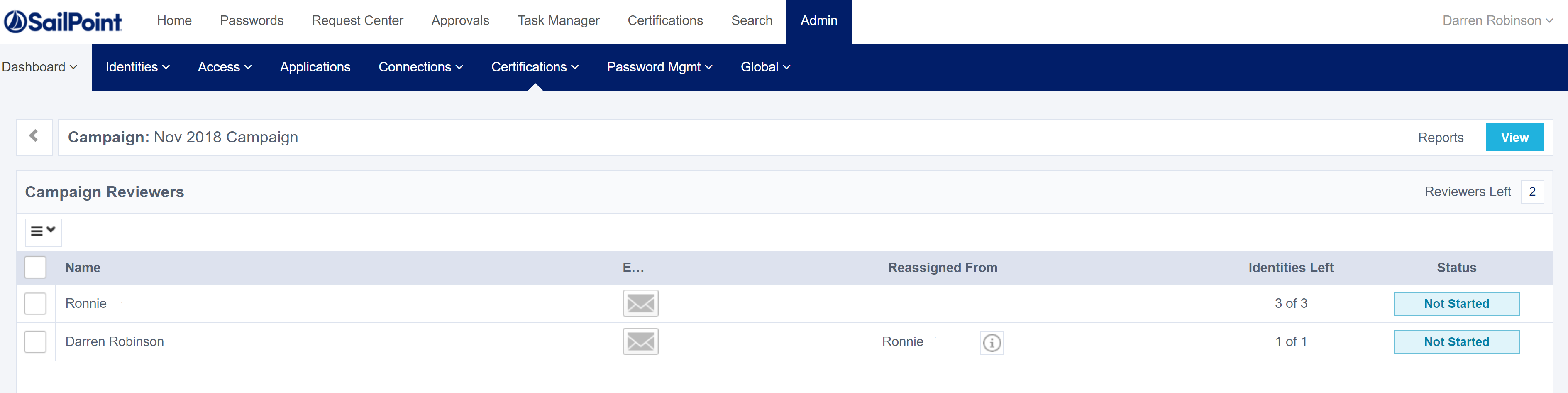
As the reviewer for Ronnie I can then go and start the review and see the Entitlements that I need to review for the campaign.
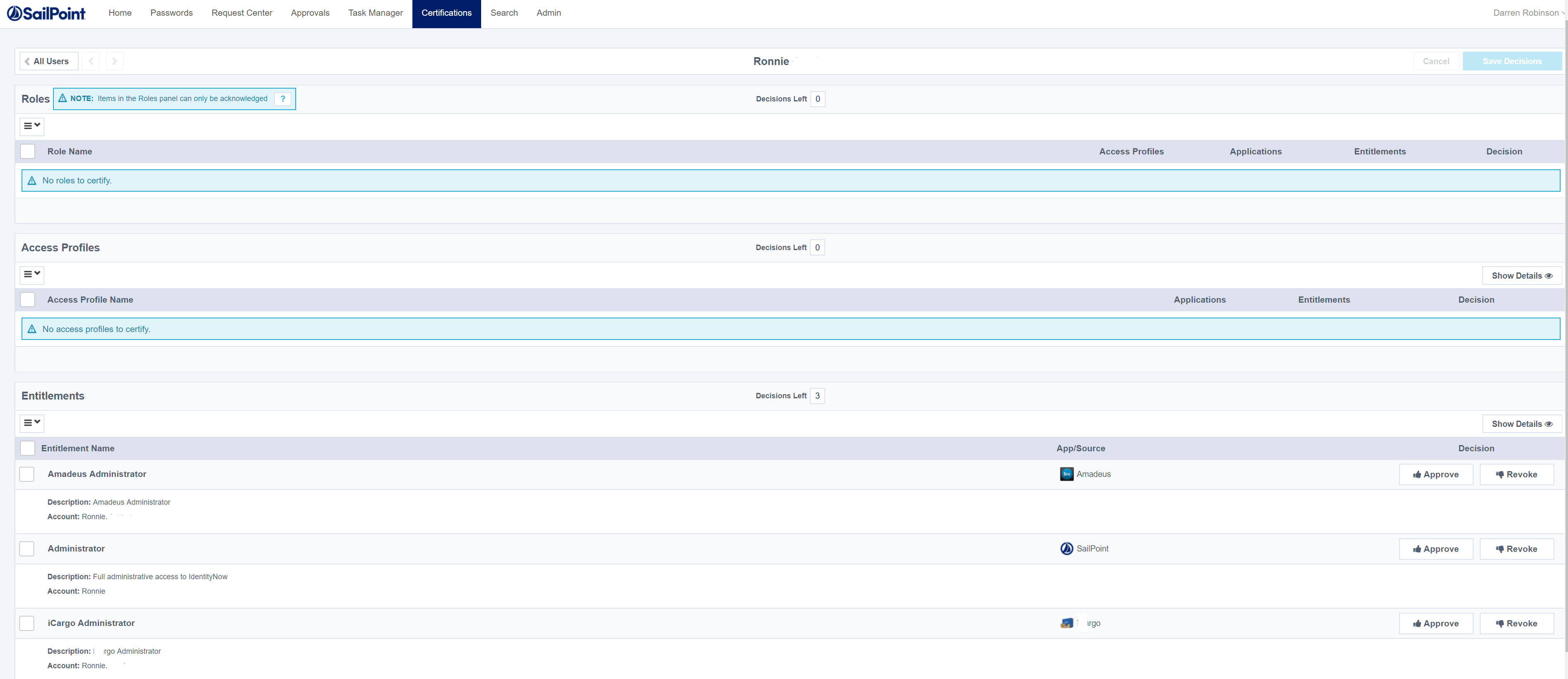
Summary
Using PowerShell we can search IdentityNow and find accounts on a Source and create a Certification Campaign for them based on Roles, Entitlements and Access Profiles. We can then also activate the campaign. Happy orchestrating.